Kotlin
Kotlin Coroutines: A Deep Dive into Modern Concurrency
Quest Lab Team • November 21, 2024 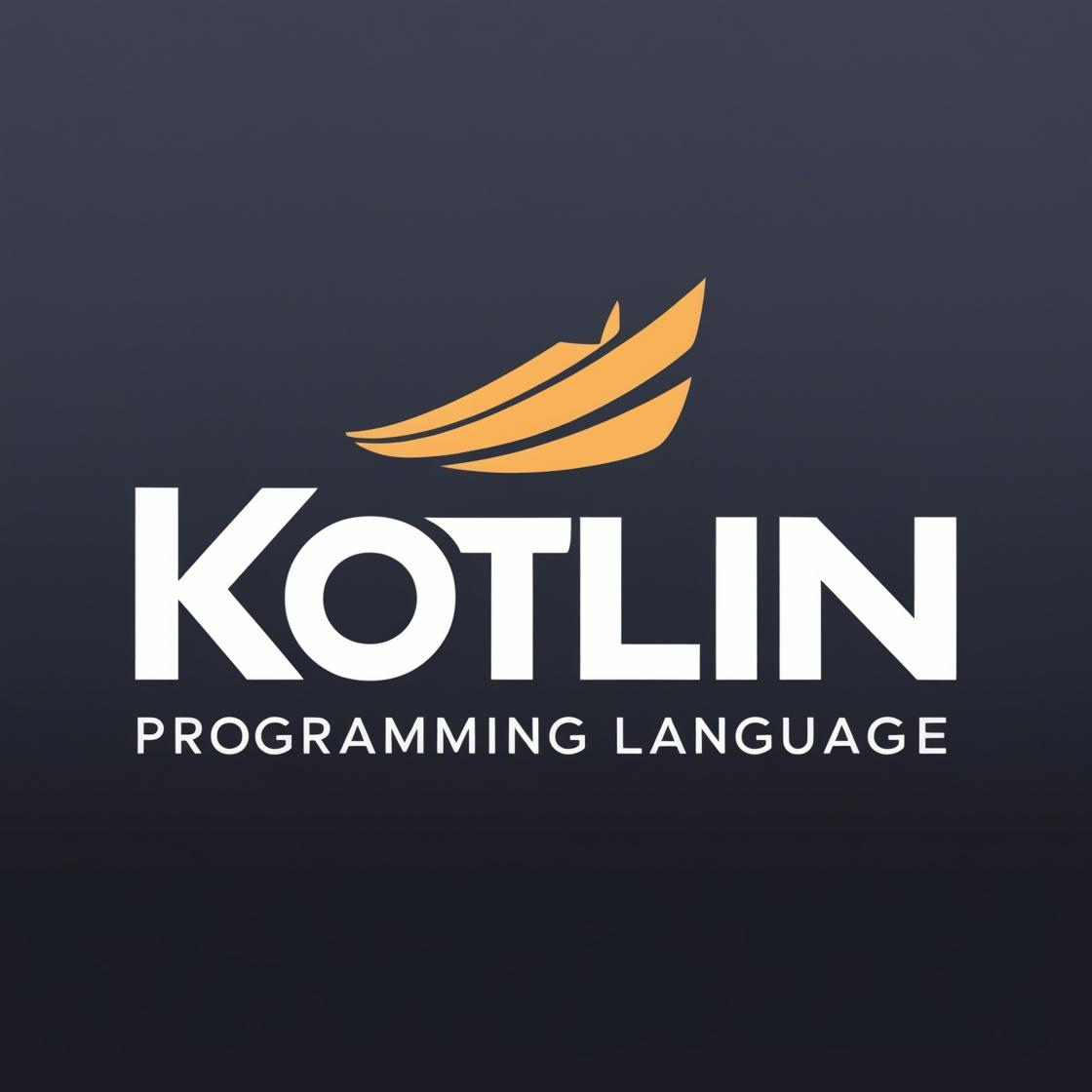
Kotlin, a modern programming language for the JVM, offerscoroutines as a powerful tool for managing concurrency. Coroutines provide a more efficient and readable alternative to traditional threads, enabling developers to write asynchronous code that appears synchronous and sequential. This article will explore the intricacies of Kotlin coroutines, examining their core features, benefits, and practical applications.
Beyond Threads: The Need for Coroutines
Concurrency, the ability to execute multiple tasks seemingly at the same time, is essential for modern applications. Threads have been the conventional approach to concurrency on the JVM, but they come with inherent complexities. Managing threads can lead to code that is difficult to understand, debug, and maintain, particularly as the number of concurrent operations increases.
Kotlin coroutines address these challenges by providing alightweight and intuitive mechanism for managing concurrency. Coroutines are not threads themselves but rather a way to manage the execution of code within threads. They achieve this through the concept ofsuspension , which allows a function to pause its execution without blocking the underlying thread. This non-blocking behavior is critical for maintaining responsiveness and efficiency in concurrent applications.
Key Features of Kotlin Coroutines
Several key features underpin the power and flexibility of Kotlin coroutines:
- Suspend Functions: Declared with the suspend keyword, these functions can be paused and resumed, enabling non-blocking execution. When a suspend function is called, it can execute a portion of its code, suspend its execution, and then resume from the same point later, without blocking the thread.
- Coroutine Scope: Coroutines must be launched within a CoroutineScope, which manages their lifecycle and execution context. The scope defines the boundaries of the coroutine's execution and handles aspects like error propagation and cancellation.
- Structured Concurrency: Kotlin coroutines promote structured concurrency, which ensures that child coroutines complete before their parent. This structured approach simplifies error handling and resource management, as the parent coroutine is responsible for the completion of its children.
- Launch Keyword: The launch keyword starts a new coroutine within a specified CoroutineScope. This allows developers to initiate concurrent operations within a well-defined context.
Simplifying Class Declarations with Kotlin
Beyond coroutines, Kotlin streamlines the declaration and manipulation of classes compared to Java. Kotlin emphasizes conciseness and readability, allowing developers to define classes and their members with minimal boilerplate code. For instance,data classes in Kotlin automatically generate common methods like equals and toString, reducing the amount of repetitive code.
Kotlin also introduces the concept offunction extensions , allowing developers to add new functions to existing classes, even those from external libraries. This feature provides greater flexibility in extending the functionality of classes without modifying their original source code.
Illustrating Coroutines in Action: A Simple Example
This example demonstrates how to use Kotlin Coroutines to perform a long-running task in the background without blocking the main thread. The code shows how to launch a coroutine to fetch data and update the UI upon completion.
This example demonstrates structured concurrency using a coroutine scope. It shows how to group multiple coroutines and handle their lifecycle together, ensuring proper cleanup if needed.
Let's break down this example:
Practical Advantages of Utilizing Coroutines
- Simplified Concurrency: Coroutines abstract away the complexities of thread management, making concurrent code easier to write and understand. They provide a higher-level abstraction that allows developers to focus on the logic of their concurrent operations rather than the low-level details of thread synchronization and communication.
- Improved Readability: Coroutines allow developers to express asynchronous operations in a sequential, synchronous style, enhancing code readability. This makes it easier to reason about the flow of execution and understand the interactions between different parts of the code.
- Enhanced Performance: By avoiding thread blocking, coroutines improve application responsiveness and efficiency. Suspending a coroutine does not consume thread resources, unlike blocking a thread. This allows applications to handle a larger number of concurrent operations without performance degradation.
- Structured Error Handling: Structured concurrency ensures proper error propagation and handling within coroutine hierarchies. If an error occurs in a child coroutine, it is propagated up to the parent coroutine, which can then handle the error appropriately.
- Cross-Platform Compatibility: Coroutines work seamlessly across various platforms and compilation targets supported by Kotlin. This makes them a versatile choice for developing concurrent applications that can run on different environments, including Android, backend servers, and web applications.
Practical Uses of Coroutines
- Parallelizing Computations: Coroutines can efficiently execute tasks in parallel and aggregate their results. This is useful for tasks that can be broken down into smaller, independent units of work, such as processing large datasets or performing complex calculations.
- UI Updates: In applications with user interfaces, coroutines can perform background tasks while keeping the UI responsive. By offloading long-running operations to coroutines, the UI thread can remain free to handle user interactions, ensuring a smooth and responsive user experience.
- Network Operations: Coroutines are well-suited for handling asynchronous network requests and responses. They can manage the sending and receiving of data over the network without blocking the main thread, allowing the application to continue processing other tasks while waiting for network operations to complete.
- Database Interactions: Coroutines can manage database queries without blocking the main thread. This is particularly important in applications that rely heavily on database access, as it prevents the UI from freezing while waiting for database operations to finish.
Kotlin coroutines offer a powerful and elegant approach to managing concurrency. They simplify the development of concurrent applications by providing a lightweight, non-blocking mechanism that enhances code readability, improves performance, and ensures structured error handling. By mastering coroutines, Kotlin developers can unlock the full potential of concurrent programming and build highly responsive and efficient applications.
Quest Lab Writer Team
This article was made live by Quest Lab Team of writers and expertise in field of searching and exploring
rich technological content on Web development and its impacts in the modern world