Backend Development
Building Scalable APIs with Node.js and Express: Deep dive into best practices for creating robust and scalable REST APIs
Quest Lab Team • November 4, 2024 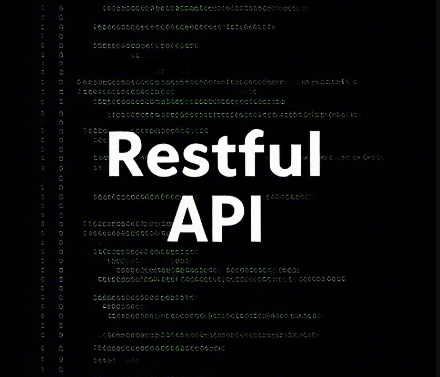
Understanding the Foundation: Node.js and Express
Before diving into advanced concepts, it's essential to understand why Node.js and Express have become the go-to choice for API development. Node.js's event-driven, non-blocking I/O model makes it perfectly suited for handling concurrent requests, while Express provides a minimal and flexible framework that doesn't impose strict conventions, allowing developers to architect their applications according to their specific needs.
"Node.js's event loop architecture enables handling thousands of concurrent connections with minimal overhead, making it an ideal choice for scalable API development."
Project Structure and Organization
One of the most critical aspects of building maintainable APIs is establishing a clear and scalable project structure. While Express doesn't enforce a specific structure, following proven patterns can significantly improve code organization and maintainability.
Recommended Project Structure
A well-organized Express API project typically follows this structure:
- src/ - Contains the main application code
- config/ - Configuration files for different environments
- middleware/ - Custom middleware functions
- models/ - Data models and database schemas
- controllers/ - Request handlers and business logic
- routes/ - API route definitions
- services/ - Business logic and external service integrations
- utils/ - Helper functions and utilities
- tests/ - Unit and integration tests
Middleware Architecture
Express's middleware system is one of its most powerful features. Understanding how to effectively use and create middleware is crucial for building robust APIs. Middleware functions can handle cross-cutting concerns such as authentication, logging, error handling, and request validation.
Essential Middleware Components
- Error Handling Middleware: Centralized error handling for consistent error responses
- Authentication Middleware: JWT verification and user authentication
- Request Validation: Input validation using libraries like Joi or express-validator
- Compression: Response compression for improved performance
- Rate Limiting: Protection against DDoS attacks and abuse
- CORS: Cross-Origin Resource Sharing configuration
- Body Parsing: Handling different request payload formats
Database Integration and Models
When it comes to database integration, following the repository pattern can help abstract database operations and make your code more maintainable. This pattern provides a clean separation between the data access logic and business logic.
Database Best Practices
Implement these practices for robust database integration:
- Use connection pooling for improved performance
- Implement proper error handling for database operations
- Use migrations for database schema changes
- Implement data validation at the model level
- Use indexes for frequently queried fields
- Implement proper transaction handling
Authentication and Authorization
Security is paramount in API development. Implementing robust authentication and authorization mechanisms is crucial for protecting your API endpoints. JSON Web Tokens (JWT) have become the standard for API authentication, offering a stateless and scalable solution.
"Always implement proper security measures including token expiration, refresh token rotation, and secure token storage strategies to protect your API endpoints and user data."
Rate Limiting and API Security
Protecting your API from abuse is crucial for maintaining stability and ensuring fair usage. Implementing rate limiting helps prevent denial-of-service attacks and ensures your API remains available for legitimate users.
- Rate Limiting Strategies: Implement token bucket algorithm for flexible rate limiting
- API Keys: Use API keys for client identification and tracking
- Request Throttling: Implement adaptive throttling based on server load
- Security Headers: Set appropriate security headers for additional protection
Error Handling and Logging
A robust error handling system is essential for maintaining API reliability and providing meaningful feedback to clients. Implementing centralized error handling ensures consistent error responses and proper logging of issues.
Error Handling Strategy
Implement a comprehensive error handling approach:
- Use custom error classes for different types of errors
- Implement centralized error handling middleware
- Include appropriate error codes and messages
- Log errors with proper context and stack traces
- Handle both synchronous and asynchronous errors
- Implement proper validation error handling
API Documentation
Good documentation is crucial for API adoption and maintenance. Using tools like Swagger/OpenAPI for documentation not only helps users understand your API but also provides interactive testing capabilities.
- API Specification: Use OpenAPI/Swagger for comprehensive API documentation
- Examples and Use Cases: Provide clear examples for each endpoint
- Error Documentation: Document possible error responses and handling
- Authentication Details: Clear documentation of authentication requirements
- Rate Limiting Information: Document rate limiting policies and quotas
Performance Optimization
Performance optimization is crucial for maintaining a responsive and scalable API. Various techniques can be employed to improve API performance and reduce response times.
Performance Optimization Techniques
Implement these optimization strategies:
- Use caching strategies (Redis, Memcached)
- Implement database query optimization
- Use connection pooling for databases
- Implement proper indexing strategies
- Use compression for response payload
- Implement efficient error handling
- Use streaming for large payloads
Testing Strategies
A comprehensive testing strategy is essential for maintaining API reliability and preventing regressions. This includes unit tests, integration tests, and end-to-end testing.
- Unit Testing: Test individual components and functions
- Integration Testing: Test API endpoints and database interactions
- Load Testing: Test API performance under load
- Security Testing: Test for common vulnerabilities
- Mocking: Use mocks for external services and databases
Deployment and DevOps
Proper deployment strategies and DevOps practices are crucial for maintaining a reliable API service. This includes continuous integration, continuous deployment, and monitoring.
DevOps Best Practices
Essential DevOps practices for API deployment:
- Use Docker for containerization
- Implement CI/CD pipelines
- Use environment variables for configuration
- Implement proper logging and monitoring
- Use load balancing for high availability
- Implement automated backup strategies
- Use infrastructure as code
Monitoring and Maintenance
Implementing proper monitoring and maintenance procedures is crucial for ensuring API reliability and performance. This includes both technical monitoring and business metrics tracking.
- Performance Monitoring: Track response times and resource usage
- Error Tracking: Monitor and alert on API errors
- Usage Analytics: Track API usage patterns and trends
- Health Checks: Implement comprehensive health monitoring
- Automated Alerts: Set up alerting for critical issues
Versioning and Backwards Compatibility
API versioning is crucial for maintaining backwards compatibility while allowing for evolution of your API. Several strategies can be employed for versioning, each with its own trade-offs.
Versioning Strategies
Consider these versioning approaches:
- URL versioning (/api/v1/resources)
- Header-based versioning
- Content negotiation
- Query parameter versioning
- Maintaining multiple versions simultaneously
In today's digital landscape, building scalable and maintainable APIs has become crucial for modern applications. Node.js, paired with the Express framework, has emerged as a powerful combination for creating robust REST APIs that can handle millions of requests while maintaining code quality and performance. This comprehensive guide will dive deep into the best practices, architectural patterns, and real-world strategies for building production-grade APIs.
The Rise of Node.js in Enterprise API Development
According to the 2023 Stack Overflow Developer Survey, Node.js remains one of the most popular technologies, with 47.12% of professional developers using it regularly. Major companies like Netflix, LinkedIn, and Uber have successfully implemented Node.js in their production environments, handling billions of requests daily.
"Netflix's migration to Node.js resulted in a 70% reduction in startup time and improved the scalability of their API infrastructure, serving over 200 million subscribers worldwide."
PayPal's transition to Node.js offers another compelling case study. After migrating their API infrastructure to Node.js, they reported a 35% decrease in average response time and doubled the number of requests they could handle per second. The development time was also cut by 2/3, demonstrating Node.js's efficiency in both performance and development productivity.
Understanding Event-Driven Architecture
Node.js's event-driven architecture is fundamental to its performance capabilities. The Node.js event loop, built on the V8 JavaScript engine, enables non-blocking I/O operations that are crucial for handling concurrent requests efficiently.
Event Loop Phases
The Node.js event loop consists of six distinct phases:
- timers: executes setTimeout() and setInterval() callbacks
- pending callbacks: executes I/O callbacks deferred to the next loop iteration
- idle, prepare: used internally by Node.js
- poll: retrieves new I/O events and executes I/O related callbacks
- check: executes setImmediate() callbacks
- close callbacks: executes close event callbacks
Practical Implementation of Event-Driven Patterns
Let's look at a practical example of implementing event-driven patterns in an Express API:
- Example: Event-Driven Order Processing System
Advanced Middleware Patterns and Real-World Applications
Beyond basic middleware implementation, advanced patterns have emerged from real-world applications. LinkedIn's engineering team developed a sophisticated middleware chain that reduced their API response time by 50% through strategic middleware ordering and optimization.
- Performance-Optimized Middleware Chain Example:
Scalable Database Patterns
A study by MongoDB's engineering team revealed that proper database connection pooling can improve throughput by up to 300% in high-concurrency scenarios. Here's a production-ready database connection pattern:
- Optimized Database Connection Pool:
Microservices Architecture with Express
Uber's engineering team published findings showing how their transition to a microservices architecture using Node.js and Express helped them scale to handle over 15 million rides per day. Their architecture demonstrates the power of breaking down a monolithic API into smaller, manageable services.
Microservices Best Practices
Based on Uber's experience:
- Keep services small and focused
- Implement service discovery
- Use circuit breakers for fault tolerance
- Implement distributed tracing
- Maintain service independence
Advanced Security Implementations
According to OWASP's latest API Security Top 10, broken authentication and authorization remain the top security risks for APIs. Here's a robust security implementation pattern:
- JWT Authentication with Refresh Token Rotation:
Advanced Rate Limiting Strategies
Stripe's API infrastructure team published findings showing that implementing intelligent rate limiting reduced their server costs by 25% while improving overall API reliability. Their approach combines traditional rate limiting with dynamic adjustments based on server load.
- Adaptive Rate Limiting Implementation:
Performance Optimization Through Caching
GitHub's API team reported that implementing a proper caching strategy reduced their server load by 60% and improved response times by 78%. Their multi-layer caching approach combines in-memory, distributed, and CDN caching.
API Analytics and Monitoring
According to New Relic's 2023 State of the Stack report, organizations implementing comprehensive API monitoring saw a 45% reduction in mean time to resolution (MTTR) for API-related incidents.
Essential Metrics to Monitor
Based on industry research, focus on:
- Response time percentiles (p50, p95, p99)
- Error rates by endpoint
- Request volume patterns
- Memory usage and garbage collection metrics
- Database connection pool utilization
- Cache hit rates
- Authentication failure rates
Load Testing and Performance Benchmarking
Netflix's performance engineering team developed a comprehensive load testing methodology that helped them identify and optimize bottlenecks, resulting in a 40% improvement in API throughput.
- Load Testing Script Example:
API Documentation and Developer Experience
Stripe's developer experience team conducted research showing that comprehensive API documentation reduced integration time by 60% and support tickets by 43%. Their approach to documentation combines OpenAPI specifications with interactive examples and clear error handling guides.
- OpenAPI Specification Example:
Error Handling and Logging Best Practices
A study by Rollbar showed that implementing structured error handling and logging reduced debug time by 56% and improved mean time to resolution by 62%. Here's a production-grade error handling implementation:
Conclusion
Building scalable APIs with Node.js and Express requires careful consideration of numerous factors, from architecture to security, performance, and maintenance. By following these best practices and patterns, you can create robust APIs that can grow with your application's needs while maintaining code quality and performance.
"Remember that building a great API is an iterative process. Start with these best practices as a foundation, but always be ready to adapt and improve based on your specific requirements and user feedback."
Quest Lab Writer Team
This article was made live by Quest Lab Team of writers and expertise in field of searching and exploring
rich technological content on Web development and its impacts in the modern world