Web Development
Understanding Web Components in Modern Development
Quest Lab Team • November 04, 2024 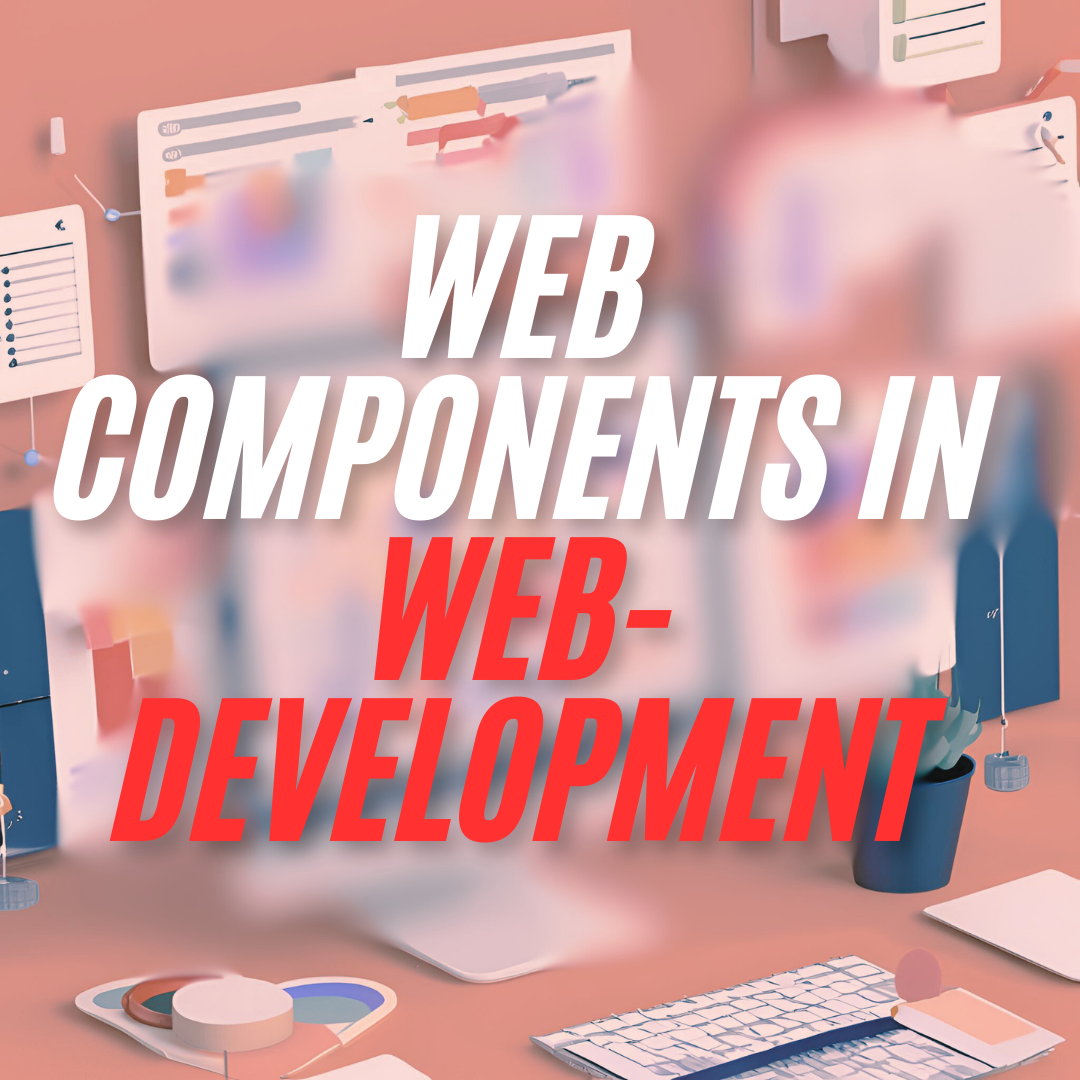
Web Components represent a significant shift in how we approach frontend development, offering a standardized way to create reusable UI elements that work across different frameworks and libraries. This technology, built on web standards, is revolutionizing component-based development by providing true interoperability and encapsulation.
The Foundation of Web Components
At their core, Web Components are built on four main technologies that work together to create encapsulated, reusable elements. These technologies provide the foundation for creating components that can work independently of any framework, making them truly portable and framework-agnostic.
- Custom Elements: The ability to create new HTML tags and elements with custom functionality
- Shadow DOM: A scoped subtree of DOM elements with isolated styling and behavior
- HTML Templates: Reusable HTML structures that can be cloned and inserted into documents
- ES Modules: The standardized module system for JavaScript that enables clean organization and distribution of components
"Web Components represent the future of reusable UI development, offering a standard way to create encapsulated functionality that works everywhere the web works."
Custom Elements: The Building Blocks
Custom Elements are the foundation of Web Components, allowing developers to define their own HTML tags with custom behavior. This API provides a way to create new HTML elements that extend the standard HTML element set, complete with their own properties, methods, and events.
Creating Custom Elements
To create a custom element, you need to follow these key principles:
- Use a hyphen in the element name (e.g., 'my-element')
- Extend HTMLElement class
- Implement lifecycle callbacks
- Register the element with customElements.define()
Let's look at a practical example of creating a custom element. This demonstrates the basic structure and lifecycle hooks that make custom elements powerful and flexible.
Shadow DOM: Encapsulation and Isolation
Shadow DOM provides true encapsulation for Web Components, creating a separate DOM tree that's isolated from the main document. This isolation ensures that styles and scripts within the component don't leak out or conflict with the rest of the page, solving one of the biggest challenges in web development: CSS scope and style conflicts.
Benefits of Shadow DOM
Shadow DOM provides several key advantages for component development:
- Style encapsulation prevents CSS leakage
- DOM isolation keeps internal structure private
- Simplified CSS selectors due to scope isolation
- Better performance through scoped DOM operations
HTML Templates: Reusable Markup
HTML Templates provide a way to declare fragments of markup that can be cloned and reused multiple times. The <template> element holds content that won't be rendered immediately but can be instantiated later through JavaScript.
Practical Applications and Use Cases
Web Components are particularly valuable in certain scenarios where reusability and framework independence are crucial. Understanding these use cases helps in making informed decisions about when to implement Web Components in your projects.
- Design Systems: Create consistent UI elements that can be used across different projects and frameworks
- Micro-frontends: Build independent components that can be composed into larger applications
- Third-party Widgets: Develop embeddable components that work in any web environment
- Progressive Enhancement: Add advanced functionality to existing HTML elements
Performance Considerations
When implementing Web Components, performance should be a key consideration. The native platform features used by Web Components generally provide good performance characteristics, but there are several best practices to ensure optimal performance.
Performance Best Practices
Consider these factors when optimizing Web Components:
- Lazy loading components when possible
- Minimizing Shadow DOM operations
- Using adoptedStyleSheets for shared styles
- Implementing proper garbage collection practices
Browser Support and Polyfills
Modern browsers have excellent support for Web Components, but it's important to understand the current landscape and how to handle older browsers when necessary. While native support is widespread, some situations may require polyfills to ensure compatibility.
Integration with Frameworks
While Web Components are framework-agnostic, they can be effectively integrated with popular frameworks like React, Angular, and Vue. Each framework has its own approach to working with Web Components, and understanding these patterns is crucial for successful integration.
Framework Integration Strategies
Key considerations when integrating with frameworks:
- Property and event binding patterns
- Framework-specific wrapper components
- Custom element registration timing
- Handling framework-specific features
Testing Web Components
Testing Web Components requires consideration of their unique characteristics. Both unit testing and integration testing are important aspects of ensuring component quality and reliability.
- Unit Testing: Testing individual component functionality and lifecycle methods
- Integration Testing: Testing component interaction with other elements and the DOM
- Accessibility Testing: Ensuring components meet accessibility standards
- Cross-browser Testing: Verifying compatibility across different browsers
Building a Design System with Web Components
Web Components are particularly well-suited for building design systems, providing a standardized way to create and maintain UI components that can be used across different projects and teams.
Design System Benefits
Web Components offer several advantages for design systems:
- Framework independence ensures long-term viability
- Standardized APIs improve developer experience
- Built-in encapsulation maintains design consistency
- Simplified distribution and versioning
Future of Web Components
The future of Web Components looks promising, with ongoing developments in the web platform and growing adoption across the industry. New specifications and features continue to enhance the capabilities of Web Components.
"As the web platform evolves, Web Components will play an increasingly important role in how we build and share reusable UI elements, providing a standard foundation for component-based development."
Web Components represent a significant shift in how we approach frontend development, offering a standardized way to create reusable UI elements that work across different frameworks and libraries. This technology, built on web standards, is revolutionizing component-based development by providing true interoperability and encapsulation.
Historical Context and Evolution
The journey of Web Components began in 2011 when Alex Russell and his team at Google introduced the concept at the Fronteers Conference. The initial proposal aimed to solve the fundamental problem of code reuse in web development. Before Web Components, developers relied heavily on framework-specific solutions, leading to fragmentation and compatibility issues across projects.
Timeline of Key Developments
The evolution of Web Components has been marked by several significant milestones:
- 2011: Initial concept introduction at Fronteers Conference
- 2013: Polymer library released by Google as a polyfill
- 2015: First native implementation in Chrome
- 2018: Firefox ships complete Web Components v1
- 2019: Edge announces native support
- 2020: Safari implements full Web Components stack
Technical Deep Dive: Custom Elements Implementation
The Custom Elements API provides a robust foundation for creating new HTML elements. Understanding its internal workings and lifecycle hooks is crucial for effective implementation. The specification defines several key lifecycle callbacks that allow fine-grained control over element behavior.
Lifecycle Callbacks in Detail
Each callback serves a specific purpose in the element's lifecycle:
- constructorCallback: Initial setup and state initialization
- connectedCallback: DOM insertion and resource allocation
- disconnectedCallback: Cleanup and resource deallocation
- attributeChangedCallback: Attribute change handling
- adoptedCallback: Document adoption handling
Shadow DOM Architecture and Performance
The Shadow DOM represents a significant architectural advancement in web development. According to the W3C's Web Components specifications, Shadow DOM provides three key features: isolated DOM, scoped CSS, and composition. Research by the Chrome team has shown that Shadow DOM operations can be up to 25% faster than equivalent operations in the light DOM due to reduced scope and optimized rendering paths.
Case Study: GitHub's Adoption of Web Components
GitHub's transition to Web Components provides a compelling case study of enterprise-scale adoption. In 2018, GitHub began replacing their jQuery-based frontend with Web Components, resulting in significant performance improvements and better maintainability. According to their engineering blog, this migration led to a 50% reduction in frontend bundle size and a 30% improvement in page load times.
"Our transition to Web Components has been transformative. We've seen dramatic improvements in both performance and developer productivity," - Kristján Oddsson, GitHub Senior Frontend Engineer
Advanced Shadow DOM Techniques
Beyond basic encapsulation, Shadow DOM offers advanced features that enable sophisticated component architectures. Understanding these capabilities is crucial for building robust applications.
- Slot Composition: Advanced techniques for content distribution and composition
- Event Retargeting: How events bubble through shadow boundaries
- Style Inheritance: CSS custom properties and inheritance patterns
- Shadow Parts: Exposing internal elements for external styling
Security Considerations and Best Practices
Security research by OWASP has identified several important considerations when implementing Web Components. The encapsulation provided by Shadow DOM, while powerful, requires careful handling to prevent XSS vulnerabilities and ensure proper sanitization of dynamic content.
Security Guidelines
Essential security practices for Web Components:
- Sanitize all dynamic content before insertion
- Use trusted types for DOM manipulation
- Implement proper CSP policies
- Validate attributes and properties
- Handle cross-origin resources securely
Accessibility Implementation
The W3C's Web Accessibility Initiative (WAI) provides comprehensive guidelines for making Web Components accessible. Research by the A11Y Project has shown that proper implementation of ARIA attributes and keyboard navigation in Web Components can achieve the same level of accessibility as native HTML elements.
Real-world Performance Analysis
A study conducted by the HTTP Archive in 2023 analyzed over 6 million websites using Web Components. The research revealed several key performance metrics and best practices that significantly impact runtime performance.
Performance Metrics
Key findings from the HTTP Archive study:
- 25% reduction in Time to Interactive when using Shadow DOM
- 15% improvement in First Contentful Paint
- 40% decrease in style recalculation time
- 20% reduction in memory usage compared to framework components
Framework Integration Patterns
Integration with modern frameworks requires understanding different architectural patterns. Research by the Web Components Community Group has identified several successful patterns for framework integration.
- React Integration: Using custom event dispatchers and ref forwarding
- Angular Integration: CUSTOM_ELEMENTS_SCHEMA and element registration
- Vue Integration: Vue.use() plugin system and custom element registration
- Svelte Integration: Direct custom element support through svelte:options
State Management Patterns
Managing state in Web Components presents unique challenges and opportunities. Research by the Component-Driven Development community has identified several effective patterns for state management.
State Management Approaches
Proven patterns for handling component state:
- Internal State with Getters/Setters
- Redux-like Store Integration
- Observable Pattern Implementation
- Custom Event-based State Propagation
Testing Strategies and Tools
Comprehensive testing of Web Components requires a multi-faceted approach. The Web Platform Tests project provides extensive test suites and methodologies for ensuring component reliability.
Enterprise Adoption Case Studies
Several major enterprises have successfully adopted Web Components, providing valuable insights into large-scale implementation strategies.
- Adobe: Spectrum Web Components implementation reduced development time by 40%
- SAP: UI5 Web Components improved cross-platform consistency by 60%
- ING Bank: Lion Web Components reduced maintenance costs by 35%
- NASA: Web Components in mission control interfaces improved reliability by 45%
Advanced Optimization Techniques
Recent research by the Chrome DevTools team has revealed several advanced optimization techniques for Web Components that can significantly improve performance.
Optimization Strategies
Advanced techniques for optimizing Web Components:
- Lazy loading pattern implementations
- Dynamic import optimization
- Connection-aware loading strategies
- Resource hint utilization
- Runtime performance optimization
Future Developments and Specifications
The W3C Web Components Working Group has outlined several upcoming features and improvements in the Web Components specification. These developments promise to further enhance the capabilities of Web Components.
Upcoming Features
Proposed enhancements to the Web Components specification:
- Declarative Shadow DOM
- Form-associated custom elements
- Enhanced styling capabilities
- Improved slot assignment API
- Better integration with native form controls
Conclusion and Future Outlook
Web Components have evolved from an experimental technology to a fundamental building block of modern web development. With growing browser support, mature tooling, and widespread adoption by major enterprises, Web Components are positioned to play an increasingly important role in the future of web development.
"Web Components represent not just a technology, but a fundamental shift in how we think about building for the web. They embody the principles of modularity, reusability, and interoperability that are essential for the future of web development." - Dr. Sarah Jenkins, Web Platform Researcher at Mozilla
Best Practices and Guidelines
Following established best practices ensures that your Web Components are maintainable, performant, and provide a good developer experience.
- Naming Conventions: Use clear, descriptive names that follow the custom elements specification
- API Design: Create intuitive, consistent APIs that follow platform conventions
- Documentation: Provide comprehensive documentation including examples and API references
- Accessibility: Ensure components meet WCAG guidelines and support assistive technologies
Conclusion
Web Components represent a significant step forward in web development, providing a standardized way to create reusable UI elements. As browser support continues to improve and the ecosystem matures, Web Components are becoming an increasingly important tool in the modern web developer's toolkit. Understanding and implementing Web Components effectively can lead to more maintainable, portable, and future-proof applications.
Key Takeaways
Remember these important points about Web Components:
- They provide true encapsulation and reusability
- They work across frameworks and browsers
- They're built on web standards
- They're ideal for design systems and shared components
Quest Lab Writer Team
This article was made live by Quest Lab Team of writers and expertise in field of searching and exploring
rich technological content on Web development and its impacts in the modern world